Laravel, presentation of the PHP web framework
Laravel, a renowned PHP development framework, is much more than just a tool for web developers. Offering a neat code structure, well-defined templates such as the MVC model, and a range of powerful features, Laravel shines for its flexibility, adapting to both modest projects and large-scale enterprises.
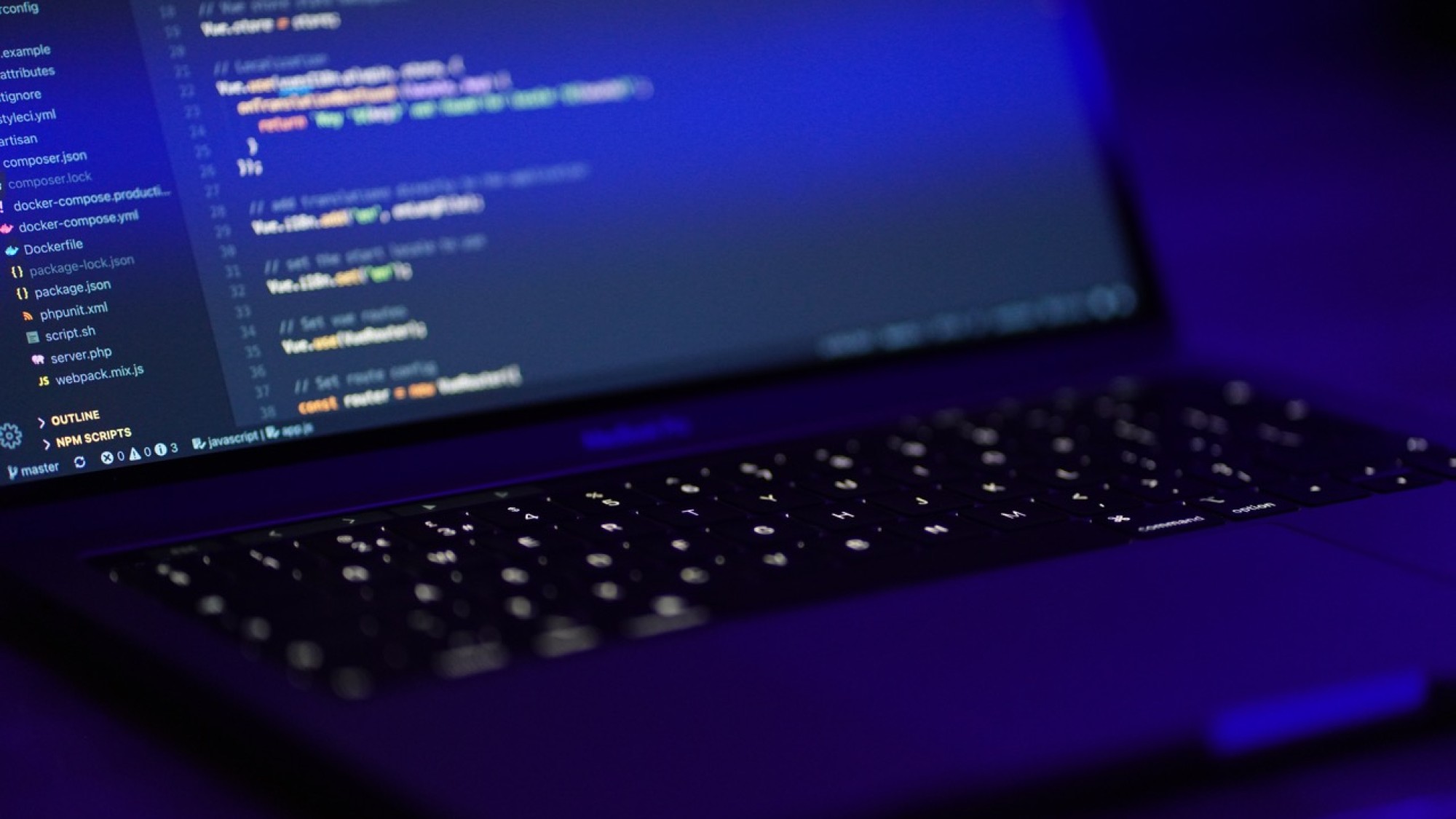
What is Laravel?
In a nutshell, the PHP Framework Laravel is a development environment offering a set of tools, for web artisans, made for developers. There are many PHP frameworks out there, but what makes Laravel better? The power of Laravel lies in the readability of the code: the code is well structured and a PHP class rarely exceeds 500 lines. The framework uses the MVC (model, view, controller) model as its basis and relies on many other implementation patterns, such as the Provider pattern and the Repository pattern.
Thanks to these well-defined patterns, Laravel is equally well suited to large and small projects. There are also a number of useful help functions.
Why use Laravel for your web projects?
Laravel is a powerful, easy-to-use PHP web framework that offers a variety of integrated features for creating websites and web apps. Here's a list of the main advantages that make Laravel a relevant choice for your projects:
- The artisans commands, a command-line tool for generating migrations, templates, controllers and much more. You can also create your own commands.
- The routes, all routes are in routes/web.php. Most of the time, we'll be writing routes that point to a controller action.
//routes/web.php
Route::get( ’user/profile’, [UserProfileController::class, ’show’])->name(’profile’);
// Generating URLs ...
$url = route(’profile’);
// Generating Redirects ...
return redirect()->route(’profile’);
- Dependency injections, as the name suggests, allow us to inject dependencies directly. For example, if we have a BookRepository that we want to use in our controller, we can inject it directly into the constructor, and it will be resolved by Laravel's service provider at runtime.
class BookController extends Controller
{
private $repository;
public function __construct(BookRepository $repository)
{
$this->repository = $repository;
}
...
}
- Middleware, a mechanism for filtering incoming requests and giving context to incoming requests, such as authenticated users.
- Databases: there are migrations to create our tables, seeders to insert data and the query builder to write our database queries.
//easily build queries
$user = DB::table(’users’)->where(’name’, ’John’)->first();
//paginate
DB::table(’users’)->paginate(15);
- The models allow us to define all our relationships and manage attributes (or add new ones) with a system of mutators (a method for modifying and controlling a value) with getters and setters . Returns an Eloquent object or collection.
- Eloquent collections with its array of array manipulation functions.
$collection = collect([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]);
//chain different methods
$sequence = $collection
->chunk(2)
->mapSpread(function (int $even, int $odd) {
return $even + $odd;
})
->all();
// [1, 5, 9, 13, 17]
- Queues are used to process slower tasks in the background. For example, if sending an e-mail takes a long time, you can put it in the queue as a job and it will be executed in the background by the server, without blocking the user with a long request.
- The vues with Blade template system to generate HTML pages. It is also possible to easily integrate JS frameworks such as VueJs and build SPAs (single page app). A translation system is also available to easily create pages in different languages.
Laravel also integrates broadcasting, caching, session and authentication systems.
And if basic functionality isn't enough for you, there are a growing number of official packages. For example, Horizon, a queue manager that offers a dashboard for managing all tasks in the queue. Different authentication systems: Sanctum, an authentication system for apis, Socialite for OAuth authentication, Breeze for basic authentication, or Jetstream for a more complete system with forgotten passwords, team management, etc. Different development environments with Sail (docker images), Homestead (virtual machine) or Valet (locally installed nginx server).
Laravel's strengths
You'll always find an elegant way to write your code with Laravel. And if we get stuck, the community is huge and always ready to help. Often I even find the solution myself by browsing Laravel's source code, which is very well written and documented. Its creator, Taylor Otwell, has a real eye for detail, and nothing is left to chance. In fact, if you look closely, all comments in the Laravel source code are formatted in the same way, and always on 3 lines.
One of Laravel's strong points is how fast and easy it is to create websites. Thanks to the wide range of built-in functionalities, complex and sophisticated websites can be created quickly and efficiently. What's more, we can easily extend Laravel's built-in functionality to meet the specific needs of our app.
Laravel is also known for its security. The framework offers a variety of security mechanisms such as protection against SQL injections and XSS (Cross-site scripting) attacks.
Laravel's weaknesses
If you're looking for high-performance websocketing, Laravel may not be your best ally. Laravel can't receive websocket messages, so you'll need to make an API call that can then broadcast to the websocket. And with each API call, you have to instantiate Laravel, which takes a bit of time. Unlike a websocket server implemented in JavaScript, which can process incoming messages directly.
The bootstrap, with each request, Laravel is primed, which can have a impact on performance. There are best practices for reducing this bootstrap time. In production, don't leave the debug (APP_DEBUG=true), as our app is exposed to security risks. Caching config, routes and views is also a good practice not to be neglected.
The final word
In conclusion, Laravel is a solid framework thanks to its wide range of integrated features, its large, active community and its security. It allows you to write elegant, easy-to-maintain code for both small and large projects. A very good stack to start a project with is Tall (TailwindCSS, AlpinJS, Laravel and Livewire) with TailwindCSS a CSS framework that we like a lot at Atipik, AlpineJs a lightweight JavaScript framework, Laravel which we presented here and Livewire for building dynamic interfaces. We'll be presenting these tools in future blog posts.